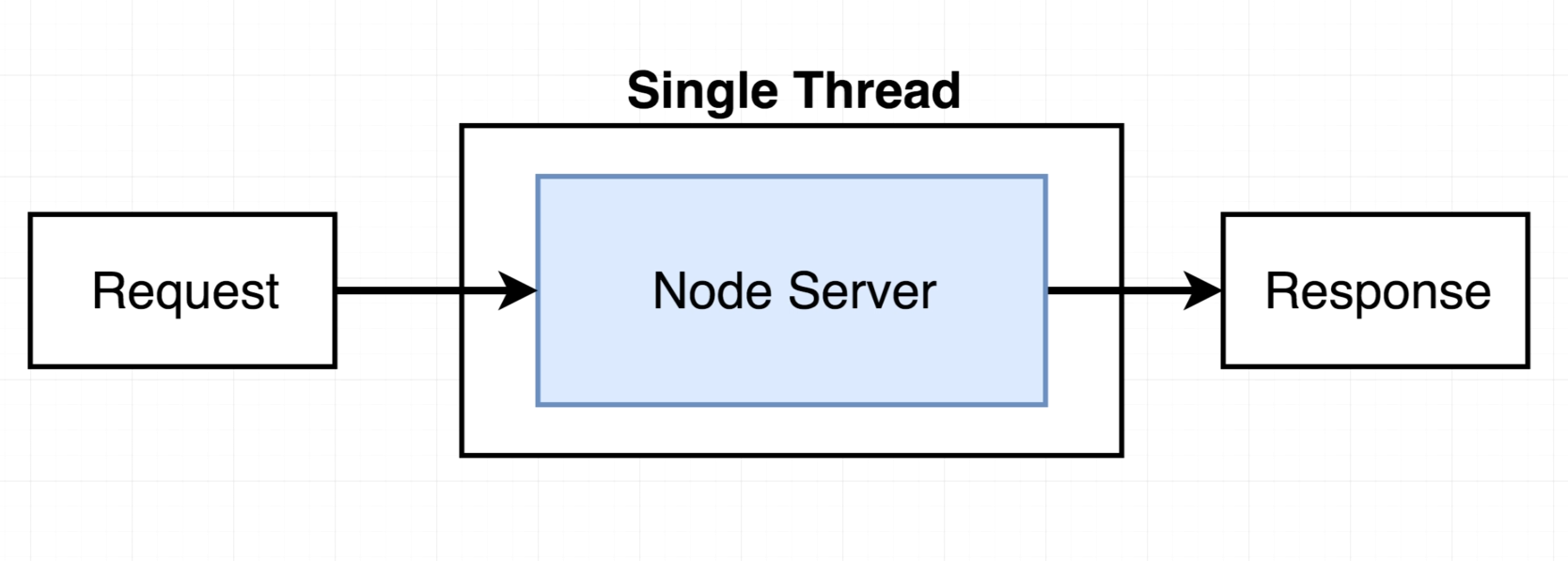
As we know, every Node.js file instance is a single thread, and has a single event loop. So to handle all the requests, it will be a bottleneck. Especially when we have a lot of requests that need much time to be processed.
Let's have a look at some examples
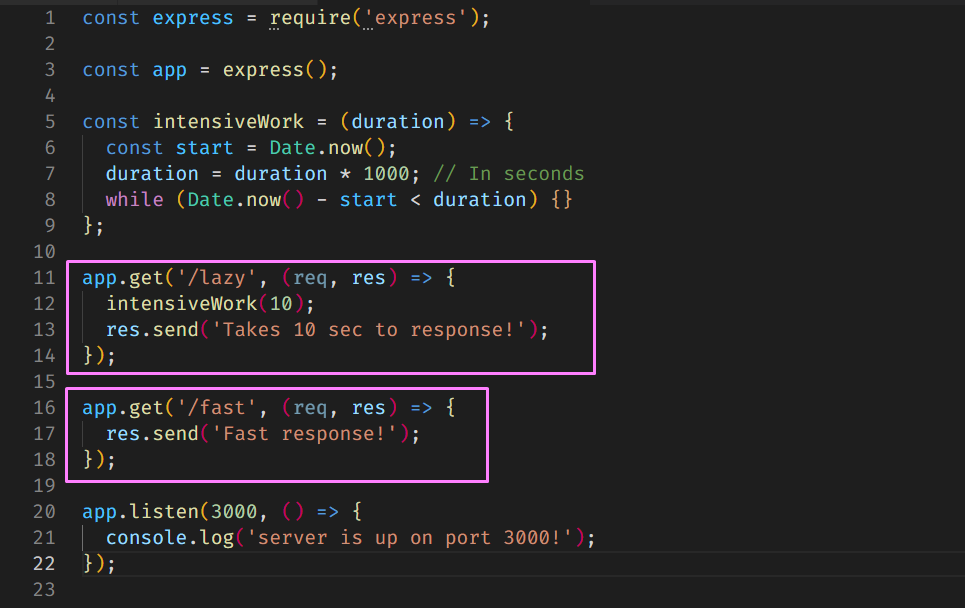
In this example, we have two routes, one of them is a slower route that returns a string after 10 seconds (lazy), and the other one is a faster route that returns a string (fast). So, when we send a request to the first route (lazy), it will be handled after 10 seconds., but when we send a request to the second route (fast), it will be handled immediately as we expect.
Lazy route (time): ⌛
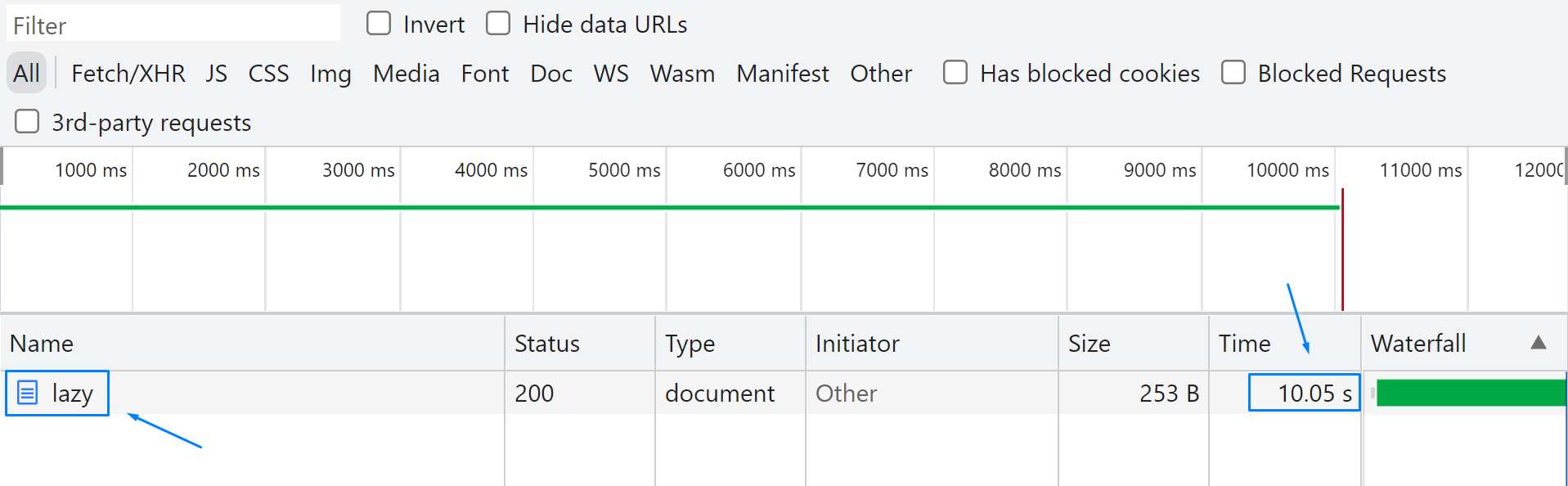
Fast route (time): ⌛
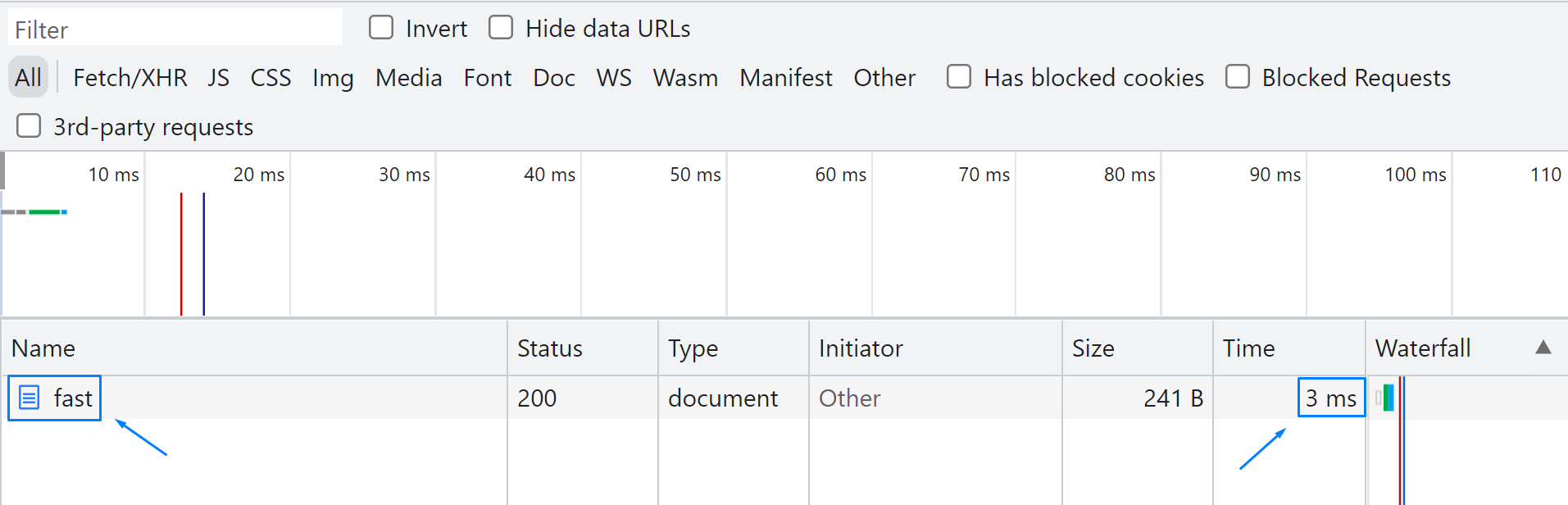
As we can see, the first route (lazy) took 10 seconds to return a string, but the second route (fast) took less than a second to return a string.
Let's try to send (lazy) route then (fast) route after it directly, what do you expect ?
Lazy route (time): ⌛
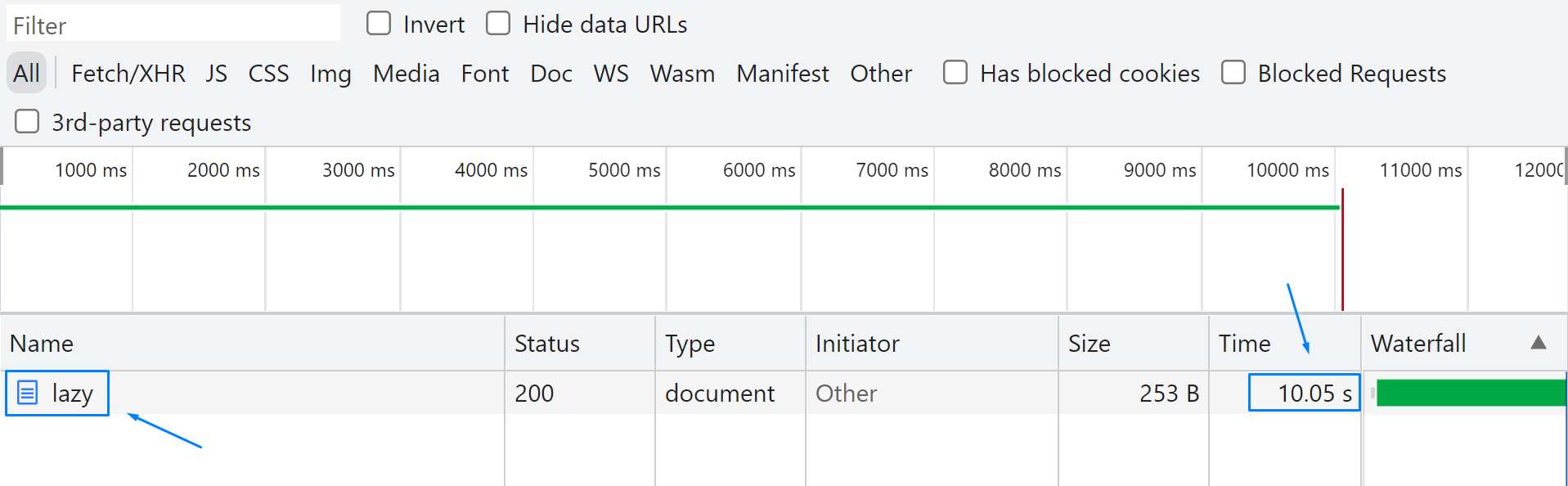
Fast route (time): ⌛
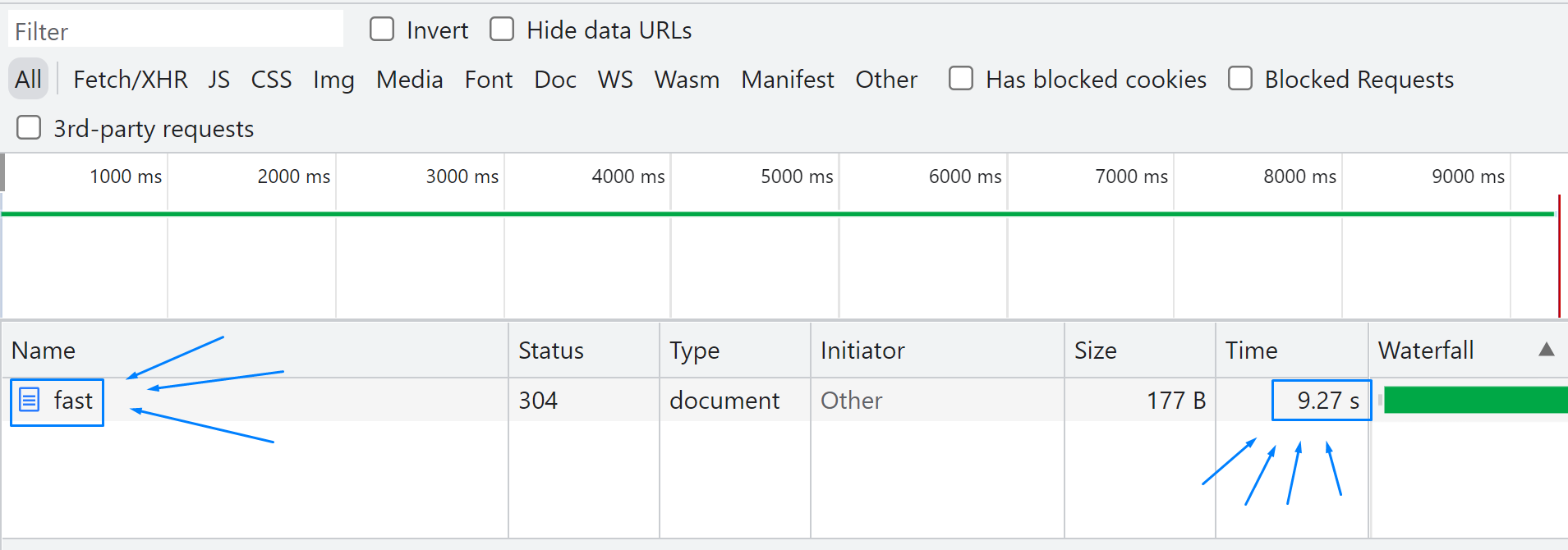
As we can see, the second route (fast) took around 10 seconds to return a string, which means it was handled after the first route (lazy). so we have a problem here that we need to solve.
What if we sent 1000 requests of lazy route at the same time ?
Let's say we have a Node.js file that has a function that takes 10 seconds to be processed. And we have 1000 requests that need to be processed. So, the first request will be processed, and the second request will be processed after 10 seconds, and the third request will be processed after 20 seconds, and so on. So, the last request will be processed after 10000 seconds, which is 166 minutes 😲.
Here's the cluster role come to play 😊
The cluster module allows us to create child processes that all share server ports. So, we can create a cluster of processes that will handle all the requests, and each process will be a single thread. So, we can handle all the requests at the same time, and we don't need to wait for other requests to be processed.
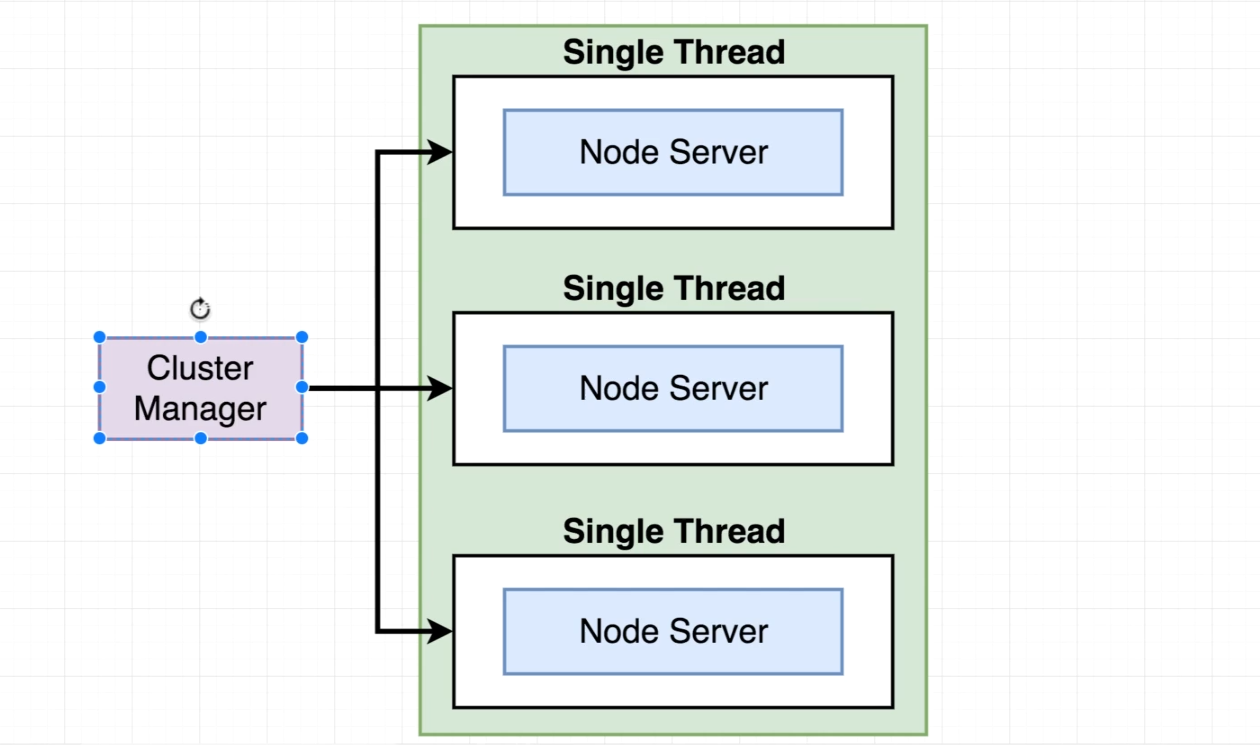
In this example, we have a cluster of 3 processes, and imagine you have 3 requests that need to be processed. So, the first request will be processed by the first process, and the second request will be processed by the second process, and the third request will be processed by the third process. So, all the requests will be processed at the same time, and we don't need to wait for other requests to be processed.
Before go through cluster let's see how node file runs:
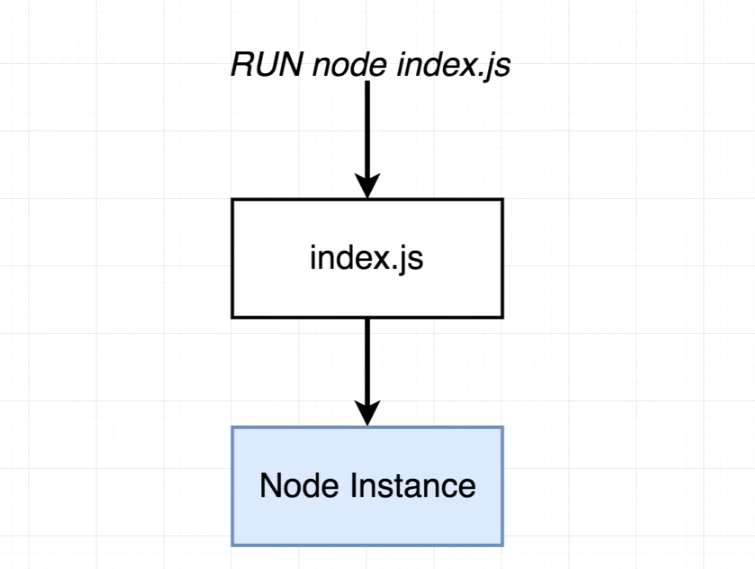
Cluster flow:
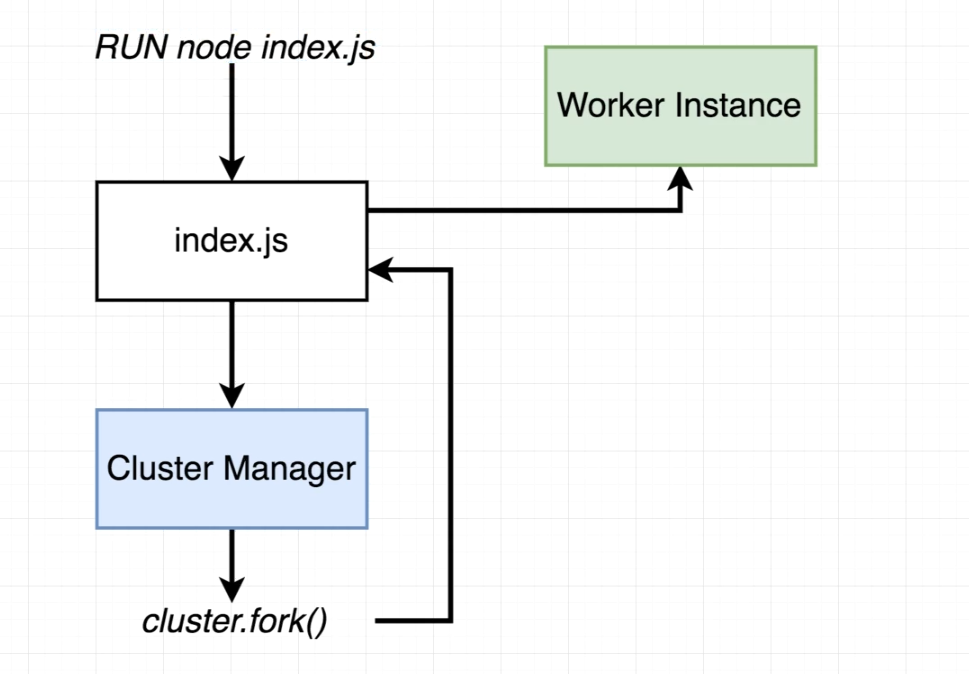
Cluster manager is the main process, and it will create a new process for each CPU core (later on). So, we can create a cluster of processes that will handle all the requests, and each process will be a single thread. So, we can handle all the requests at the same time, and we don't need to wait for other requests to be processed. and worker instance is a single thread that will handle all the requests. When we call cluster.fork() node internally goes back to index.js file and execute it again, but this time it will be a child process. so index.html file will be executed multiple times first time will produce master process (Cluster Manager) and the rest will produce worker instances.
Enough theory ! 👋
Let's add cluster to our code 📌
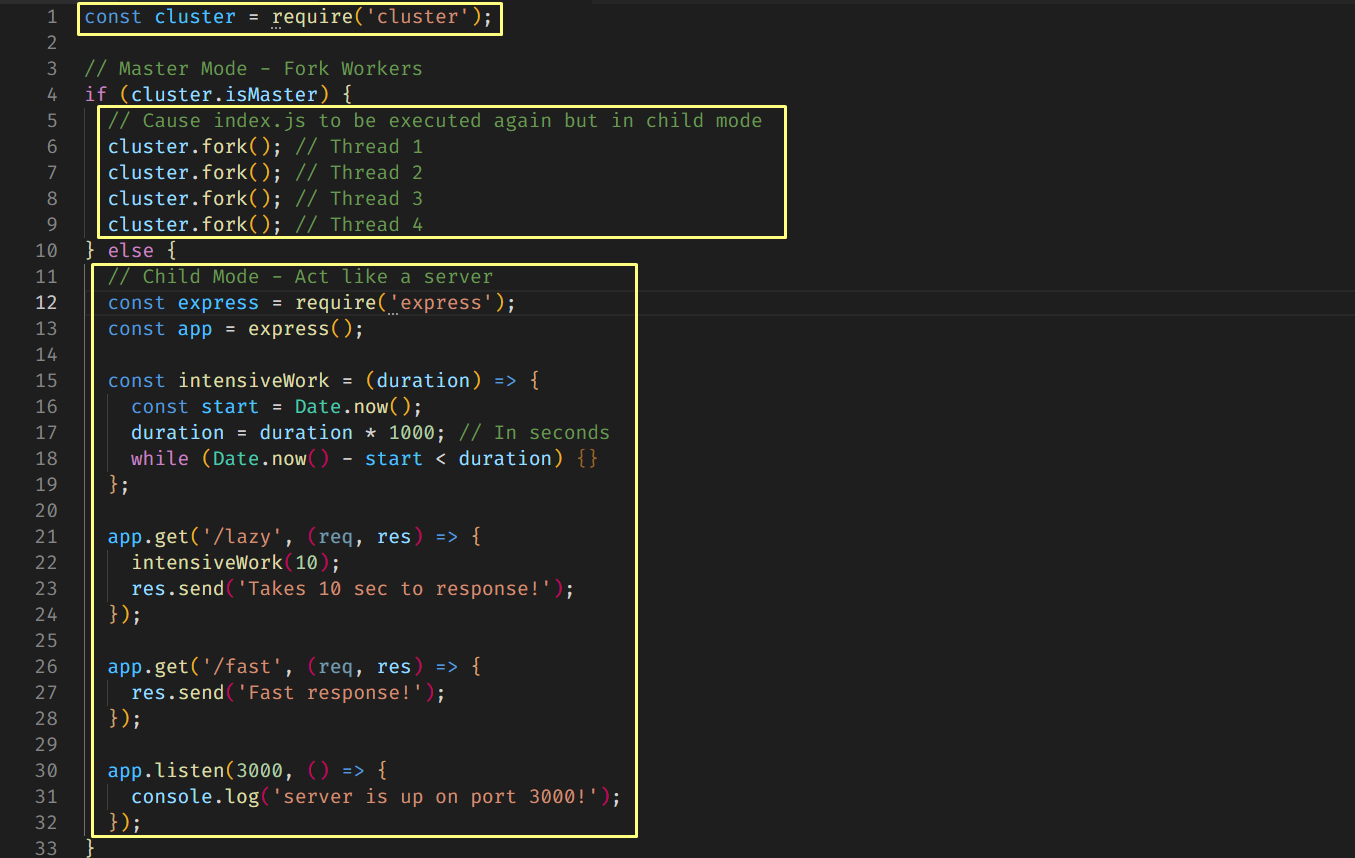
The first thing you will notice is that our server has been called 4 times, and we have 4 processes. as apears in the pic down.

Now we about to end this article, but before that, let's call our 2 routes (lazy then fast) and see what happens.
Fast route (before): ❎
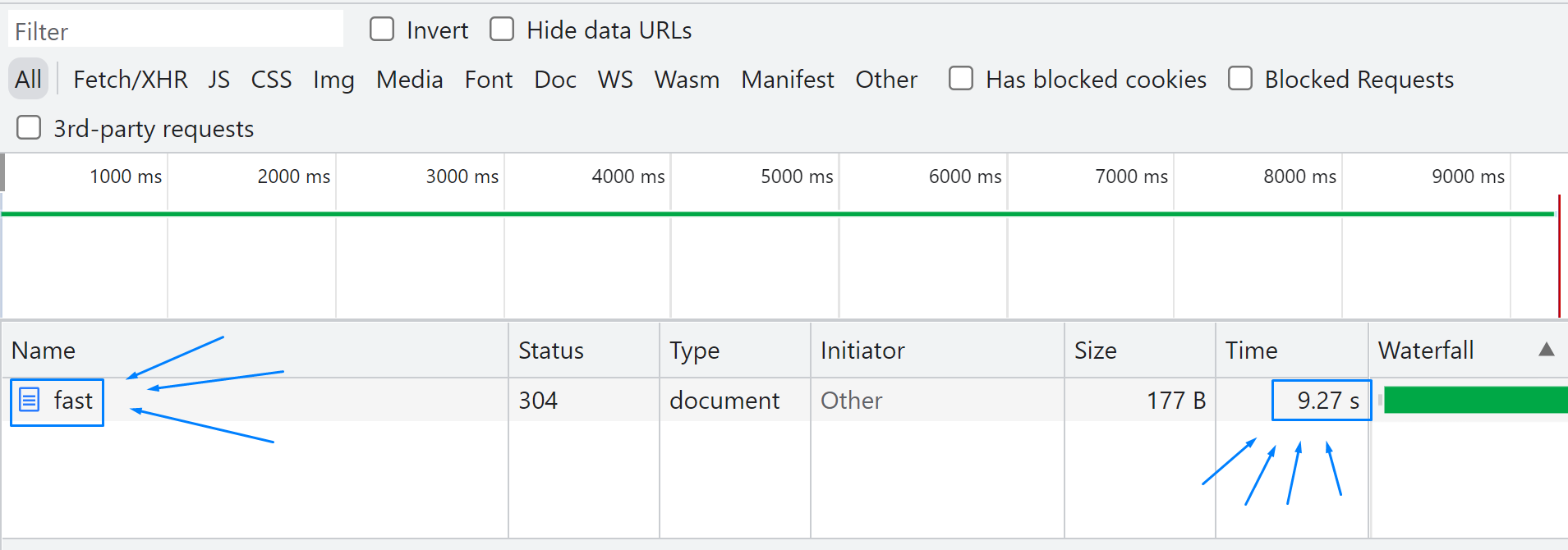
Fast route (after): ✅
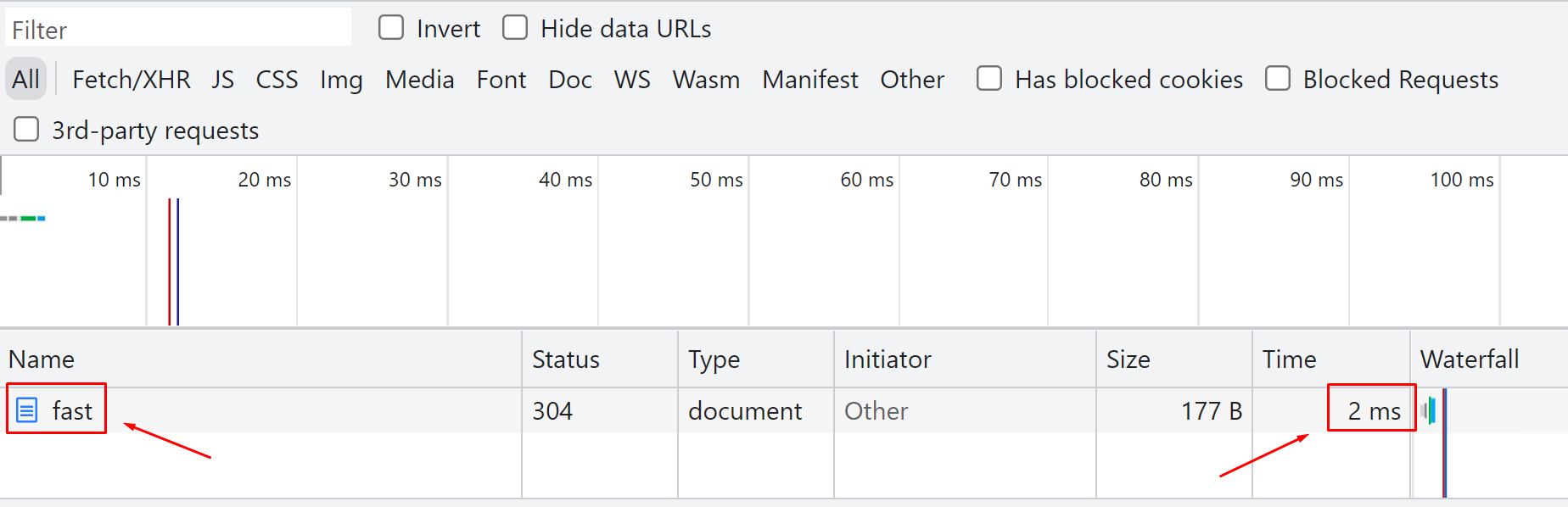
Conclusion:
As we saw we can handle all the requests at the same time, and we don't need to wait for other requests to be processed. It's like we have 4 servers that handle all the requests at the same time. BUT Cluster has a problem, it has some limitations, and it's not suitable for all cases. So, we need to know when to use it and when not to use it. Try to use PM2 instead of native cluster, it's more powerful and it has more features.
Leave a Reply